Top ReactJS Interview Questions and Answers for 2024
Outline – Top ReactJS Interview Questions
- Introduction
- Importance of ReactJS in 2024
- Overview of the Article
- Basic ReactJS Questions
- What is ReactJS?
- What are the features of ReactJS?
- Explain the virtual DOM.
- What is JSX?
- ReactJS Components
- What are components in React?
- Difference between class and functional components
- Explain props in React.
- What is the state in React?
- Lifecycle Methods
- What are React lifecycle methods?
- Explain componentDidMount() and componentWillUnmount().
- Hooks in React
- What are hooks?
- Explain useState hook.
- What is useEffect hook?
- Difference between useState and useRef
- Event Handling in React
- How do you handle events in React?
- Explain the concept of synthetic events in React.
- How to pass a value to an event handler?
- React Router
- What is React Router?
- Explain the difference between BrowserRouter and HashRouter.
- How to implement nested routes?
- State Management
- What is state management in React?
- Explain Redux.
- Difference between Redux and Context API
- Styling in React
- How to style components in React?
- What are styled-components?
- Difference between CSS modules and styled-components
- Advanced Topics
- What is server-side rendering (SSR)?
- Explain code splitting.
- What is lazy loading in React?
- Testing in React
- How to test React components?
- What is Jest?
- Explain the use of Enzyme in React testing.
- Performance Optimization
- How to optimize the performance of a React app?
- Explain memoization in React.
- What is React.memo?
- React Best Practices
- What are the best practices for React development?
- How to structure React projects?
- Importance of key prop in lists
- React and TypeScript
- How to use TypeScript with React?
- Benefits of using TypeScript in React projects
- Difference between PropTypes and TypeScript
- Conclusion
- Summary of key points
- Final thoughts on preparing for a ReactJS interview
- FAQs
- What is the future of ReactJS?
- How to stay updated with ReactJS?
- What are the common mistakes in ReactJS development?
- How important is it to know Redux for a React job?
- Can ReactJS be used for mobile app development?
Introduction
In the ever-evolving landscape of web development, ReactJS continues to dominate as a powerful and popular JavaScript library for building user interfaces. As we move into 2024, the demand for skilled React developers remains high. Whether you’re a seasoned developer or just starting your journey, preparing for ReactJS interview questions is crucial. This article compiles the top 50 ReactJS interview questions and answers to help you succeed in your next interview.
Basic ReactJS Questions
What is ReactJS?
ReactJS is an open-source JavaScript library developed by Facebook for building dynamic user interfaces, particularly single-page applications. It allows developers to create reusable UI components and manage the state efficiently.
What are the features of ReactJS?
ReactJS boasts several features, including:
- Component-based architecture
- Virtual DOM
- JSX (JavaScript XML)
- Unidirectional data flow
- High performance and speed
Explain the virtual DOM.
The virtual DOM is a lightweight representation of the actual DOM. When the state of an object changes, the virtual DOM updates only that object in the real DOM, rather than updating all objects, resulting in faster and more efficient rendering.
What is JSX?
JSX stands for JavaScript XML. It is a syntax extension for JavaScript that allows developers to write HTML-like code within JavaScript. JSX makes it easier to create and render React components.
ReactJS Components
What are components in React?
Components are the building blocks of a React application. They encapsulate logic and UI elements, making it easier to manage and reuse code. Components can be either class components or functional components.
Difference between class and functional components
Class components are ES6 classes that extend from React.Component and can manage their own state and lifecycle methods. Functional components are simple JavaScript functions that receive props as arguments and return React elements. With the introduction of hooks, functional components can also manage state and lifecycle.
Explain props in React.
Props, short for properties, are read-only attributes passed from a parent component to a child component. They enable data flow between components and allow for component reusability.
What is the state in React?
State is a built-in React object used to manage dynamic data within a component. Unlike props, the state is mutable and can change over time, usually in response to user actions.
Lifecycle Methods
What are React lifecycle methods?
Lifecycle methods are special methods in React components that are invoked at different stages of a component’s life. These stages include mounting, updating, and unmounting.
Explain componentDidMount() and componentWillUnmount().
componentDidMount()
: Called immediately after a component is mounted. Ideal for initializing data or making API calls.componentWillUnmount()
: Called just before a component is unmounted and destroyed. Useful for cleanup tasks like cancelling network requests or removing event listeners.
Hooks in React
What are hooks?
Hooks are functions introduced in React 16.8 that allow functional components to use state and other React features without writing class components.
Explain useState hook.
The useState
hook allows functional components to manage state. It returns an array with two elements: the current state value and a function to update it.
What is useEffect hook?
The useEffect
hook lets you perform side effects in functional components, such as fetching data, directly manipulating the DOM, or setting up subscriptions. It combines the functionalities of componentDidMount
, componentDidUpdate
, and componentWillUnmount
.
Difference between useState and useRef
useState
: Used for creating and updating state variables.useRef
: Used for accessing and persisting a mutable value without causing re-renders.
Event Handling in React
How do you handle events in React?
Events in React are handled using event handlers, which are functions defined inside components. These handlers are attached to elements via props like onClick
, onChange
, etc.
Explain the concept of synthetic events in React.
Synthetic events are React’s cross-browser wrapper around the browser’s native event. They ensure that events have consistent properties across different browsers, improving compatibility and performance.
How to pass a value to an event handler?
You can pass values to event handlers by wrapping them in an anonymous function. For example:
<button onClick={() => handleClick(value)}>Click me</button>
React Router
What is React Router?
React Router is a library for routing in React applications. It enables navigation between different components and views, maintaining the application’s state and URL in sync.
Explain the difference between BrowserRouter and HashRouter.
BrowserRouter
: Uses the HTML5 history API to create clean and readable URLs.HashRouter
: Uses the hash portion of the URL (e.g.,www.example.com/#/home
) to simulate different pages.
How to implement nested routes?
Nested routes are implemented by defining child routes within a parent route using Route
components. This allows for complex layouts and routing hierarchies.
State Management
What is state management in React?
State management refers to the practice of managing and sharing state across different components in a React application. It ensures consistency and predictability in how state changes occur.
Explain Redux.
Redux is a state management library for JavaScript applications, including React. It follows a unidirectional data flow and helps manage the global state using actions, reducers, and a centralized store.
Difference between Redux and Context API
- Redux: Offers a more structured and scalable approach to state management with middleware support.
- Context API: Built into React, suitable for simpler state management needs without additional libraries.
Styling in React
How to style components in React?
Components in React can be styled using various methods, including CSS, inline styles, CSS-in-JS libraries like styled-components, and CSS modules.
What are styled-components?
Styled-components is a library that allows you to write CSS directly within your JavaScript code. It uses tagged template literals to style components, promoting a more modular and scoped approach.
Difference between CSS modules and styled-components
- CSS modules: Scope CSS by default, preventing conflicts by generating unique class names.
- Styled-components: Use JavaScript to create encapsulated styles, providing dynamic styling capabilities.
Advanced Topics
What is server-side rendering (SSR)?
SSR is a technique where React components are rendered on the server and sent to the client as fully rendered HTML pages. This improves initial load time and SEO performance.
Explain code splitting.
Code splitting is a technique that divides a codebase into smaller chunks or bundles, which can be loaded on demand. This improves the performance of large applications by reducing initial load time.
What is lazy loading in React?
Lazy loading is a technique to defer the loading of non-essential resources until they are needed. In React, it can be achieved using React.lazy
and Suspense
to load components dynamically.
Testing in React
How to test React components?
React components can be tested using testing libraries like Jest and Enzyme. These tools provide utilities to render components, simulate user interactions, and assert expected outcomes.
What is Jest?
Jest is a JavaScript testing framework developed by Facebook. It offers a powerful and easy-to-use API for writing and running tests for JavaScript applications, including React components. It supports features like snapshots, mocking, and coverage reporting.
Explain the use of Enzyme in React testing.
Enzyme is a JavaScript testing utility for React that makes it easier to test component output. Developed by Airbnb, Enzyme provides methods for shallow rendering, full DOM rendering, and static rendering, allowing for more granular and precise tests.
Performance Optimization
How to optimize the performance of a React app?
Performance optimization in React can be achieved through several techniques:
- Memoization: Using
React.memo
anduseMemo
to prevent unnecessary re-renders. - Code splitting: Implementing dynamic imports to load parts of the application on demand.
- Lazy loading: Deferring the loading of non-essential components until needed.
- Virtualization: Using libraries like
react-window
orreact-virtualized
for rendering large lists efficiently. - PureComponent: Extending from
React.PureComponent
for class components to perform shallow prop and state comparison.
Explain memoization in React.
Memoization is an optimization technique to cache the result of a function call and return the cached result when the same inputs occur again. In React, React.memo
and useMemo
are used to memoize components and values, reducing unnecessary re-renders and computations.
What is React.memo?
React.memo
is a higher-order component (HOC) that memoizes a functional component, preventing it from re-rendering if its props haven’t changed. This enhances performance by avoiding unnecessary renders.
React Best Practices
What are the best practices for React development?
Some best practices for React development include:
- Component composition: Break down complex UIs into smaller, reusable components.
- State management: Keep state local to where it is needed and lift state up when necessary.
- Keys in lists: Always provide a unique
key
prop for list items to maintain component identity. - PropTypes and TypeScript: Use PropTypes or TypeScript to ensure type safety and catch potential bugs early.
- Code splitting and lazy loading: Implement these techniques to improve performance and load times.
How to structure React projects?
Structuring a React project involves organizing files and folders in a way that enhances maintainability and scalability. A common structure includes:
- src: The main source folder.
- components: Reusable components.
- containers: Components connected to state or Redux.
- assets: Static assets like images and fonts.
- services: API calls and external services.
- utils: Utility functions and helpers.
- styles: Global and component-specific styles.
Importance of key prop in lists
The key
prop helps React identify which items have changed, are added, or are removed. Providing a unique key
for each list item improves performance by enabling efficient re-renders and updates.
React and TypeScript
How to use TypeScript with React?
TypeScript can be integrated into a React project by installing TypeScript and the necessary type definitions (@types/react
and @types/react-dom
). Components and files are then converted from .js
to .tsx
, and TypeScript features like interfaces, types, and generics are used to enforce type safety.
Benefits of using TypeScript in React projects
Using TypeScript in React projects offers several benefits:
- Type safety: Catching type errors during development.
- Improved developer experience: Autocompletion, better documentation, and error detection.
- Scalability: Easier to manage and refactor large codebases.
- Enhanced readability: Clear and self-documenting code.
Difference between PropTypes and TypeScript
- PropTypes: A runtime type checking mechanism for React props, used to validate props passed to components.
- TypeScript: A static type checker that catches type errors at compile-time, offering a more robust solution for type safety across the entire codebase.
Conclusion
ReactJS remains a leading choice for building dynamic and performant web applications. Preparing for a ReactJS interview requires a solid understanding of its fundamentals, advanced concepts, and best practices. By familiarizing yourself with these top 50 ReactJS interview questions and answers, you’ll be well-equipped to tackle any interview and demonstrate your expertise in React development.
FAQs
What is the future of ReactJS?
ReactJS continues to evolve with frequent updates and a strong community. It remains a popular choice for front-end development due to its flexibility, performance, and extensive ecosystem. The future of ReactJS looks promising as it adapts to new trends and technologies in web development.
How to stay updated with ReactJS?
Staying updated with ReactJS involves following official React documentation, joining community forums, subscribing to newsletters, attending conferences, and participating in online courses and tutorials. also check our more posts.
What are the common mistakes in ReactJS development?
Common mistakes in ReactJS development include:
- Mutating state directly: Always use setState or appropriate state update functions.
- Not using keys in lists: Keys are crucial for list rendering and performance.
- Improper use of lifecycle methods: Ensure correct usage to avoid bugs and performance issues.
- Overusing Redux: Use state management libraries only when necessary; sometimes Context API or local state is sufficient.
How important is it to know Redux for a React job?
Knowing Redux can be important for a React job, especially for applications requiring complex state management. However, it’s not always necessary, as other state management solutions like Context API can be suitable for simpler use cases.
Can ReactJS be used for mobile app development?
Yes, ReactJS can be used for mobile app development through React Native. React Native allows developers to build native mobile apps for iOS and Android using React and JavaScript, sharing a significant portion of the codebase between web and mobile applications.
By mastering these questions and answers, you’ll be better prepared to showcase your ReactJS knowledge and skills in any interview setting. Good luck!
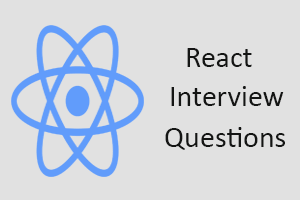
Share this page on:
Subscribe Email Updates
to get latest update